The No Nonsense Guide to Java Spring Boot
3:03:58
Description
Go from Java Developer to Backend Engineer using Java 17 + mySQL
What You'll Learn?
- Java Spring Boot
- Controller, Service, Repository layers
- Exception Handling & Reponses
- Validation
- Query String Parameters, @Query, Search
- JDBC, JPA, Hibernate, Spring Data, ORMs
- Unit Testing
- Headers
- Relational Mappings
- Logging
- Cache
- External API Integration
- Spring Security (Basic Auth)
- Spring Security (JWTs - JSON Web Tokens)
- Dependecy Injection
- Spring Boot Actuator
- Transactions & @Transactional
- Dependency Injection, Inversion of Control, Spring Container
Who is this for?
What You Need to Know?
More details
DescriptionThis comprehensive course is designed to transition Java developers into proficient backend engineers by mastering the essentials of Spring Boot. Unlike other courses that dive into complex concepts before covering the basics, this course follows a hands-on, real-world approach. We focus on building practical applications using live production code techniques, modern Java abstractions, and clear naming conventions commonly used in industry projects.
Youâll start by understanding how Spring Boot works before diving into the whyâgiving you the tools to build solid foundations before exploring advanced topics. Whether youâre new to Spring Boot or looking to solidify your backend skills, this course offers a straightforward, no-nonsense guide that covers all the essential components needed for building scalable, production-ready applications.
What You Will Learn:
Controllers, Services, Repositories, and Entities: Understand the core building blocks of Spring Boot applications, including how to set up RESTful APIs, business logic layers, and data access layers.
Database Integration with MySQL: Learn how to run MySQL queries, set up database connections, and work seamlessly with relational databases.
Exception Handling & Custom Error Responses: Master best practices for handling errors, defining custom error responses, and returning meaningful error messages in production.
Input Validation: Implement input validation to ensure data integrity, and protect against invalid requests
Spring Data JPA & Custom Queries: Explore query string parameters, custom query methods with @Query, and interact with databases using Spring Data JPA.
JDBC, JPA, and Hibernate: Learn the differences between JDBC and JPA, understand ORM (Object Relational Mapping) with Hibernate, and when to use each in your applications.
Unit Testing: Write unit tests to ensure your code works as expected, covering both service and repository layers using tools like JUnit and Mockito.
Working with HTTP Headers: Learn how to work with request and response headers, implement custom headers, and pass data effectively through API endpoints.
Relational Mappings (OneToOne, OneToMany, ManyToMany): Understand how to model real-world relationships between entities in a relational database using JPA annotations.
Logging: Implement effective logging strategies to track application behavior, debug issues, and monitor performance.
Caching for Performance Optimization: Leverage caching strategies to improve application performance and scalability, including setting up default caching and custom cache eviction policies.
External API Integration: Learn how to consume external APIs, handle authentication, and integrate third-party services into your Spring Boot application.
Spring Security: Explore the built-in security mechanisms, set up Basic Authentication, and secure your applications with JWT (JSON Web Token) for token-based authentication.
Dependency Injection & Inversion of Control (IoC): Gain a solid understanding of how Spring Boot manages dependencies and objects, ensuring modular and maintainable code.
Monitoring with Spring Boot Actuator: Enable real-time monitoring of your application, track performance metrics, and expose health and status endpoints using Spring Bootâs Actuator.
Transactions & @Transactional: Manage database transactions, understand transactional boundaries, and ensure data consistency using Springâs @Transactional annotation.
Advanced Java Concepts in Spring Boot: Delve into practical Java topics frequently used in Spring Boot applications, such as UUIDs for unique identifiers, event-driven programming, handling various return types, managing JSON data in MySQL, and implementing versioning for your APIs.
Who this course is for:
- Go from Java Developer to Backend Engineer using Spring Boot
This comprehensive course is designed to transition Java developers into proficient backend engineers by mastering the essentials of Spring Boot. Unlike other courses that dive into complex concepts before covering the basics, this course follows a hands-on, real-world approach. We focus on building practical applications using live production code techniques, modern Java abstractions, and clear naming conventions commonly used in industry projects.
Youâll start by understanding how Spring Boot works before diving into the whyâgiving you the tools to build solid foundations before exploring advanced topics. Whether youâre new to Spring Boot or looking to solidify your backend skills, this course offers a straightforward, no-nonsense guide that covers all the essential components needed for building scalable, production-ready applications.
What You Will Learn:
Controllers, Services, Repositories, and Entities: Understand the core building blocks of Spring Boot applications, including how to set up RESTful APIs, business logic layers, and data access layers.
Database Integration with MySQL: Learn how to run MySQL queries, set up database connections, and work seamlessly with relational databases.
Exception Handling & Custom Error Responses: Master best practices for handling errors, defining custom error responses, and returning meaningful error messages in production.
Input Validation: Implement input validation to ensure data integrity, and protect against invalid requests
Spring Data JPA & Custom Queries: Explore query string parameters, custom query methods with @Query, and interact with databases using Spring Data JPA.
JDBC, JPA, and Hibernate: Learn the differences between JDBC and JPA, understand ORM (Object Relational Mapping) with Hibernate, and when to use each in your applications.
Unit Testing: Write unit tests to ensure your code works as expected, covering both service and repository layers using tools like JUnit and Mockito.
Working with HTTP Headers: Learn how to work with request and response headers, implement custom headers, and pass data effectively through API endpoints.
Relational Mappings (OneToOne, OneToMany, ManyToMany): Understand how to model real-world relationships between entities in a relational database using JPA annotations.
Logging: Implement effective logging strategies to track application behavior, debug issues, and monitor performance.
Caching for Performance Optimization: Leverage caching strategies to improve application performance and scalability, including setting up default caching and custom cache eviction policies.
External API Integration: Learn how to consume external APIs, handle authentication, and integrate third-party services into your Spring Boot application.
Spring Security: Explore the built-in security mechanisms, set up Basic Authentication, and secure your applications with JWT (JSON Web Token) for token-based authentication.
Dependency Injection & Inversion of Control (IoC): Gain a solid understanding of how Spring Boot manages dependencies and objects, ensuring modular and maintainable code.
Monitoring with Spring Boot Actuator: Enable real-time monitoring of your application, track performance metrics, and expose health and status endpoints using Spring Bootâs Actuator.
Transactions & @Transactional: Manage database transactions, understand transactional boundaries, and ensure data consistency using Springâs @Transactional annotation.
Advanced Java Concepts in Spring Boot: Delve into practical Java topics frequently used in Spring Boot applications, such as UUIDs for unique identifiers, event-driven programming, handling various return types, managing JSON data in MySQL, and implementing versioning for your APIs.
Who this course is for:
- Go from Java Developer to Backend Engineer using Spring Boot
User Reviews
Rating
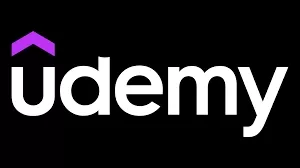
Udemy
View courses Udemy- language english
- Training sessions 82
- duration 3:03:58
- Release Date 2025/02/24