Testing Java with JUnit 5 & Mockito
Sergey Kargopolov
6:50:34
Description
Unit Testing & Integration Testing of Java and Spring Boot applications. Test Driven Development(TDD) Explained.
What You'll Learn?
- JUnit 5 - basic & advanced topics
- Test Web Layer and Controllers
- Test Java code with Mockito - the most popular Mocking Framework.
- Test Data layer and JPA Repositories
- Write Spring Boot Integration Tests
- Test Business layer and Service classes
- Master Test Driven Development (TDD)
- And more...
Who is this for?
More details
DescriptionUnit Testing is a must-have skill and this video course is about unit testing.
If you take this video course, you will learn how to test your Java code using JUnit 5 and Mockito framework.
This video course is for beginners and you do not need to have any prior Unit testing knowledge to enrol in this course.
JUnit 5 in Different Java projects
There are different Java projects, build tools and development environments. In this course, you will learn how to create a new project and configure JUnit 5 support for different types of projects, using different development environments and build tools.
You will learn how to create a Unit test in:Â
IntelliJ IDEA and
Eclipse Development environments.
You will learn how to create a Unit test in:Â
Regular Java project,
Maven-based Java project,
Cradle-based Java project.
Course Overview
This video course teaches Unit and Integration testing with Java from the very beginning and covers many advanced topics as well. By the end of this course, you will learn:
JUnit 5 basics, and
JUnit 5 advanced topics.
Once you become familiar with JUnit 5, you will learn to use:
Test-Driven Development(TDD)
You will then learn to use another very popular Test framework for Java called:Â
Mockito
You will also learn how to write:
Spring Boot integration tests
By the end of this course you will learn and be able to use all of the following:Â
Create Unit Tests in IntelliJ,
Create Unit Tests in Eclipse,
Run unit tests using Gradle,
Run Unit Tests using Maven,
Use @DisplayName annotation,
Use JUnit assertions,
Test for Exceptions,
Use Lifecycle methods (@BeforeAll, @BeforeEach, @AfterEach, @AfterAll),
Run unit tests in any order you need:Â (Random, Order by Name, Order by Index),
Disable Unit test,
Repeated Tests with @RepeatedTest annotation,
Parameterized tests with @Parameterized annotation
@ValueSource,
@MethodSource,
@CsvSource,
@CsvFileSource
Change Test Instance lifecycle with @TestInstance (PER_CLASS, PER_METHOD)
Learn to Mock objects with Mockito's @Mock annotation,
Learn to user Mockito's argument matches,
Mockito method stubbing,
Mockito Exception stubbing,
Verify method call,
Call Real Method,
Do nothing when a method is called,
Write integration tests for Spring Boot applications,
and more...
Who this course is for:
- Java developers interested in learning how to test Java applications with JUnit 5
Unit Testing is a must-have skill and this video course is about unit testing.
If you take this video course, you will learn how to test your Java code using JUnit 5 and Mockito framework.
This video course is for beginners and you do not need to have any prior Unit testing knowledge to enrol in this course.
JUnit 5 in Different Java projects
There are different Java projects, build tools and development environments. In this course, you will learn how to create a new project and configure JUnit 5 support for different types of projects, using different development environments and build tools.
You will learn how to create a Unit test in:Â
IntelliJ IDEA and
Eclipse Development environments.
You will learn how to create a Unit test in:Â
Regular Java project,
Maven-based Java project,
Cradle-based Java project.
Course Overview
This video course teaches Unit and Integration testing with Java from the very beginning and covers many advanced topics as well. By the end of this course, you will learn:
JUnit 5 basics, and
JUnit 5 advanced topics.
Once you become familiar with JUnit 5, you will learn to use:
Test-Driven Development(TDD)
You will then learn to use another very popular Test framework for Java called:Â
Mockito
You will also learn how to write:
Spring Boot integration tests
By the end of this course you will learn and be able to use all of the following:Â
Create Unit Tests in IntelliJ,
Create Unit Tests in Eclipse,
Run unit tests using Gradle,
Run Unit Tests using Maven,
Use @DisplayName annotation,
Use JUnit assertions,
Test for Exceptions,
Use Lifecycle methods (@BeforeAll, @BeforeEach, @AfterEach, @AfterAll),
Run unit tests in any order you need:Â (Random, Order by Name, Order by Index),
Disable Unit test,
Repeated Tests with @RepeatedTest annotation,
Parameterized tests with @Parameterized annotation
@ValueSource,
@MethodSource,
@CsvSource,
@CsvFileSource
Change Test Instance lifecycle with @TestInstance (PER_CLASS, PER_METHOD)
Learn to Mock objects with Mockito's @Mock annotation,
Learn to user Mockito's argument matches,
Mockito method stubbing,
Mockito Exception stubbing,
Verify method call,
Call Real Method,
Do nothing when a method is called,
Write integration tests for Spring Boot applications,
and more...
Who this course is for:
- Java developers interested in learning how to test Java applications with JUnit 5
User Reviews
Rating
Sergey Kargopolov
Instructor's Courses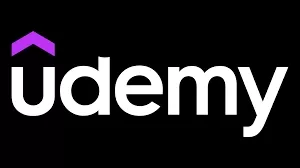
Udemy
View courses Udemy- language english
- Training sessions 96
- duration 6:50:34
- Release Date 2023/03/30