Python 3 Design Patterns Playbook
Gerald Britton
4:11:02
Description
This course will teach you how to use proven object-oriented design patterns to significantly enhance the stability, testability, and maintainability of your Python development while decreasing your development time.
What You'll Learn?
Whether you're an experienced Python developer or just getting started, having ready-to-use solutions in your toolkit will make your job easier and more satisfying. In this course, Python 3 Design Patterns Playbook, you’ll learn to effectively use object-oriented design patterns in Python. First, you’ll explore the origins of design patterns and their applicability to programming projects of all sizes. Next, you’ll discover the many design patterns described in the well-known “gang of four” design patterns book on the topic. Finally, you’ll learn how to apply these patterns to solve real-world problems that occur in business and organizations of all sizes. When you’re finished with this course, you’ll have the skills and knowledge of object-oriented Python programming needed to build stable, maintainable, and extensible applications.
More details
User Reviews
Rating
Gerald Britton
Instructor's Courses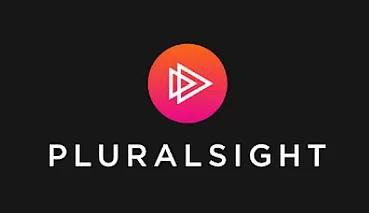
Pluralsight
View courses Pluralsight- language english
- Training sessions 141
- duration 4:11:02
- level average
- Release Date 2023/12/15