Master Data Structures and Algorithms in Python
Ravi Singh
9:57:48
Description
From Basic to Advanced Concepts, Practical Implementation and Problem Solving, Mastery of Data Structures and Algorithm
What You'll Learn?
- Advance Data Structure and Algorithms in Python : arrays, linked lists, stacks, queues, trees, and graphs
- Building a strong foundation in computer science fundamentals for efficient problem-solving
- Analyzing time and space complexity of algorithms for efficiency
- Algorithm design techniques: divide and conquer, dynamic programming, and greedy algorithms
- Using algorithmic paradigms such as brute force, backtracking, and heuristics to solve problems efficiently.
Who is this for?
What You Need to Know?
More details
DescriptionApply Coupon code, get maximum discount: 7A4A79AA47D9D9F070B7
Python is a powerful and versatile programming language, known for its simplicity and readability. This course will cover the fundamental concepts and techniques for organizing, storing, and manipulating data efficiently using Python.
The course will start with an introduction to basic data structures such as arrays, linked lists, stacks, and queues, and then move on to more complex data structures such as trees and graphs. We will explore how to implement these data structures in Python, as well as how to use them to solve real-world problems.
The course will also cover various algorithms such as sorting, searching, and graph traversal, and we will analyze the time and space complexity of these algorithms to determine their efficiency. We will explore algorithm design techniques such as divide and conquer, dynamic programming, and greedy algorithms, and we will apply these techniques to solve real-world problems.
In addition to the core data structures and algorithms, we will also cover topics such as data abstraction, complexity theory, and algorithmic paradigms such as brute force, backtracking, and heuristics. We will explore how to choose the appropriate paradigm for a given problem and how to use it to solve problems efficiently.
How data structure and algorithm course help to get placed in top tech companies?
A strong foundation in data structures and algorithms is essential for success in top tech companies, as they form the building blocks for software development. Here are some ways in which a data structure and algorithm course can help individuals get placed in top tech companies:
Problem-Solving Skills: Data structure and algorithm courses teach problem-solving skills that are essential for success in top tech companies. They provide a framework for approaching complex problems and breaking them down into smaller, more manageable tasks.
Efficiency: Top tech companies are always looking for ways to improve the efficiency of their software. Knowledge of data structures and algorithms helps individuals develop efficient programs that can handle large amounts of data quickly and reliably.
Competitive Edge: Many top tech companies look for candidates who have a strong foundation in computer science fundamentals. A data structure and algorithm course can provide individuals with a competitive edge when applying for jobs at these companies.
Technical Interviews: Technical interviews at top tech companies often focus on data structures and algorithms. A data structure and algorithm course can help individuals prepare for these interviews by giving them the necessary knowledge and practice to succeed.
Industry-Relevant Skills: A data structure and algorithm course can provide individuals with industry-relevant skills that are in high demand in top tech companies. These skills can be leveraged to stand out from other candidates and secure a position at a top tech company.
Overall, a data structure and algorithm course can help individuals develop the skills and knowledge necessary to succeed in top tech companies. It provides a strong foundation in computer science fundamentals and teaches problem-solving skills that are essential for success in the industry.
Who this course is for:
- Essential for computer science candidate to gain in-depth knowledge about data structures and algorithms
- Useful for software developers to improve skills in data storage, retrieval, and processing
- Beneficial for IT professionals to learn new skills or update their knowledge about data structures and algorithms
- Suitable for anyone with an interest in computer science and problem-solving
- Intended for individuals who want to develop a strong foundation in computer science fundamentals.
Apply Coupon code, get maximum discount: 7A4A79AA47D9D9F070B7
Python is a powerful and versatile programming language, known for its simplicity and readability. This course will cover the fundamental concepts and techniques for organizing, storing, and manipulating data efficiently using Python.
The course will start with an introduction to basic data structures such as arrays, linked lists, stacks, and queues, and then move on to more complex data structures such as trees and graphs. We will explore how to implement these data structures in Python, as well as how to use them to solve real-world problems.
The course will also cover various algorithms such as sorting, searching, and graph traversal, and we will analyze the time and space complexity of these algorithms to determine their efficiency. We will explore algorithm design techniques such as divide and conquer, dynamic programming, and greedy algorithms, and we will apply these techniques to solve real-world problems.
In addition to the core data structures and algorithms, we will also cover topics such as data abstraction, complexity theory, and algorithmic paradigms such as brute force, backtracking, and heuristics. We will explore how to choose the appropriate paradigm for a given problem and how to use it to solve problems efficiently.
How data structure and algorithm course help to get placed in top tech companies?
A strong foundation in data structures and algorithms is essential for success in top tech companies, as they form the building blocks for software development. Here are some ways in which a data structure and algorithm course can help individuals get placed in top tech companies:
Problem-Solving Skills: Data structure and algorithm courses teach problem-solving skills that are essential for success in top tech companies. They provide a framework for approaching complex problems and breaking them down into smaller, more manageable tasks.
Efficiency: Top tech companies are always looking for ways to improve the efficiency of their software. Knowledge of data structures and algorithms helps individuals develop efficient programs that can handle large amounts of data quickly and reliably.
Competitive Edge: Many top tech companies look for candidates who have a strong foundation in computer science fundamentals. A data structure and algorithm course can provide individuals with a competitive edge when applying for jobs at these companies.
Technical Interviews: Technical interviews at top tech companies often focus on data structures and algorithms. A data structure and algorithm course can help individuals prepare for these interviews by giving them the necessary knowledge and practice to succeed.
Industry-Relevant Skills: A data structure and algorithm course can provide individuals with industry-relevant skills that are in high demand in top tech companies. These skills can be leveraged to stand out from other candidates and secure a position at a top tech company.
Overall, a data structure and algorithm course can help individuals develop the skills and knowledge necessary to succeed in top tech companies. It provides a strong foundation in computer science fundamentals and teaches problem-solving skills that are essential for success in the industry.
Who this course is for:
- Essential for computer science candidate to gain in-depth knowledge about data structures and algorithms
- Useful for software developers to improve skills in data storage, retrieval, and processing
- Beneficial for IT professionals to learn new skills or update their knowledge about data structures and algorithms
- Suitable for anyone with an interest in computer science and problem-solving
- Intended for individuals who want to develop a strong foundation in computer science fundamentals.
User Reviews
Rating
Ravi Singh
Instructor's Courses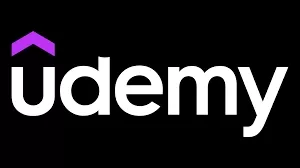
Udemy
View courses Udemy- language english
- Training sessions 62
- duration 9:57:48
- Release Date 2023/06/16