Java Programming Essentials
Focused View
10:35:57
48 View
1 - Java Programming Essentials - Introduction.mp4
04:02
2 - Module introduction.mp4
00:50
3 - Learning objectives.mp4
00:18
4 - 1.1 The Java ecosystem.mp4
06:21
5 - 1.2 Understanding Javas execution model.mp4
06:44
6 - 1.3 Understanding the value of threading and garbage collection.mp4
08:57
7 - 1.4 Understanding the value of object orientation and encapsulation.mp4
10:22
8 - Learning objectives.mp4
00:27
9 - 2.1 Creating executable Java applications.mp4
06:22
10 - 2.2 Running Java from the command line.mp4
06:22
11 - 2.3 Managing the classpath.mp4
09:27
12 - 2.4 Compiling from the command line.mp4
16:15
13 - Learning objectives.mp4
01:35
14 - 3.1 Understanding packages.mp4
07:07
15 - 3.2 Working with packages.mp4
08:42
16 - 3.3 Illustrating packages and imports.mp4
12:04
17 - 3.4 Creating packages and package-info.java.mp4
10:04
18 - Module introduction.mp4
01:00
19 - Learning objectives.mp4
00:40
20 - 4.1 Using operators, operands, and expressions.mp4
03:58
21 - 4.2 Using arithmetic operators + - %.mp4
15:35
22 - 4.3 Using the plus operator with Strings.mp4
05:02
23 - 4.4 Operand promotion in arithmetic.mp4
05:00
24 - 4.5 Using increment and decrement operators.mp4
10:27
25 - Learning objectives.mp4
00:40
26 - 5.1 Using shift operators.mp4
08:38
27 - 5.2 Using comparison operators.mp4
03:10
28 - 5.3 Using logical operators.mp4
06:03
29 - 5.4 Using short-circuit operators.mp4
05:17
30 - 5.5 Using assignment operators.mp4
09:35
31 - Learning objectives.mp4
00:29
32 - 6.1 Understanding assignment compatibility.mp4
06:49
33 - 6.2 Understanding other elements of expressions.mp4
05:24
34 - 6.3 Using parentheses and operator precedence.mp4
07:41
35 - Learning objectives.mp4
00:26
36 - 7.1 Using the == operator with primitives and references.mp4
11:04
37 - 7.2 Distinguishing == and the equals method.mp4
06:06
38 - 7.3 Discovering more about the equals method.mp4
09:53
39 - Module introduction.mp4
00:20
40 - Learning objectives.mp4
01:14
41 - 8.1 Understanding the basic form of if and if else.mp4
03:01
42 - 8.2 Using braces with if else. Effect of else if.mp4
08:07
43 - 8.3 Understanding the if else if else structure.mp4
05:40
44 - 8.4 Using the conditional operator.mp4
05:13
45 - Learning objectives.mp4
00:22
46 - 9.1 Using the general form of switch, case, break, and default.mp4
04:32
47 - 9.2 Code examples for the general form of switch.mp4
04:58
48 - 9.3 Understanding break in switch.mp4
03:48
49 - 9.4 Identifying switchable types.mp4
02:18
50 - Module introduction.mp4
00:49
51 - Learning objectives.mp4
00:22
52 - 10.1 Creating and using while loops.mp4
03:41
53 - 10.2 Code examples of the while loop.mp4
04:41
54 - 10.3 Using do while loops.mp4
02:22
55 - Learning objectives.mp4
00:31
56 - 11.1 Understanding the simple use of the for loop.mp4
05:43
57 - 11.2 Understanding the initialization section of the for loop.mp4
08:54
58 - 11.3 Understanding the test section of the for loop.mp4
01:30
59 - 11.4 Understanding the increment section of the for loop.mp4
03:07
60 - 11.5 Omitting sections of a for loop.mp4
03:17
61 - 11.6 Code examples for basic for loops.mp4
08:23
62 - Learning objectives.mp4
00:30
63 - 12.1 Using the enhanced for loop.mp4
05:18
64 - 12.2 Identifying the valid targets of the enhanced for loop.mp4
03:10
65 - 12.3 Using the enhanced for loop with generic collections.mp4
02:59
66 - 12.4 Code examples for enhanced for loops.mp4
04:41
67 - Learning objectives.mp4
00:13
68 - 13.1 Comparing while and do while loops.mp4
01:06
69 - 13.2 Comparing while and simple for loops.mp4
02:47
70 - 13.3 Comparing while and enhanced for loops over Iterables.mp4
03:36
71 - 13.4 Comparing while and enhanced for loops over arrays.mp4
03:39
72 - Learning objectives.mp4
00:29
73 - 14.1 Using break from a single loop.mp4
06:47
74 - 14.2 Using continue in a single loop.mp4
07:17
75 - 14.3 Using a labeled break in nested loops.mp4
06:10
76 - 14.4 Using a labeled continue in nested loops.mp4
03:01
77 - Module introduction.mp4
00:20
78 - Learning objectives.mp4
00:31
79 - 15.1 Understanding structure of Java source files.mp4
13:14
80 - 15.2 Understanding the structure of a Java class.mp4
08:21
81 - 15.3 Defining static methods - Part 1.mp4
10:54
82 - 15.4 Defining static methods - Part 2.mp4
13:52
83 - 15.5 Defining instance fields.mp4
14:11
84 - 15.6 Investigating mutation of objects by methods.mp4
08:02
85 - 15.7 Discovering pass-by-value.mp4
14:51
86 - 15.8 Defining instance methods - Part 1.mp4
07:22
87 - 15.9 Defining instance methods - Part 2.mp4
15:26
88 - 15.10 Initializing objects - Part 1.mp4
11:29
89 - 15.11 Initializing objects - Part 2.mp4
06:22
90 - Learning objectives.mp4
00:21
91 - 16.1 Selecting a field from a reference expression.mp4
10:58
92 - 16.2 Using this to access fields.mp4
10:38
93 - 16.3 Code examples accessing fields.mp4
07:17
94 - 16.4 Using this to access object features.mp4
04:52
95 - 16.5 Accessing static members.mp4
14:02
96 - 16.6 Scope and disambiguation of identifiers.mp4
05:57
97 - Learning objectives.mp4
00:39
98 - 17.1 Using the access modifiers public and private.mp4
05:09
99 - 17.2 Using default access and the protected modifier.mp4
05:05
100 - Learning objectives.mp4
00:31
101 - 18.1 Designing for encapsulation.mp4
05:37
102 - 18.2 Implementing encapsulation.mp4
11:26
103 - 18.3 Encapsulation code example.mp4
21:52
104 - 18.4 Implementing immutability.mp4
13:34
105 - Module introduction.mp4
00:27
106 - Learning objectives.mp4
00:38
107 - 19.1 Understanding the value and mechanism of garbage collection.mp4
05:56
108 - 19.2 Collecting garbage.mp4
11:18
109 - Java Programming Essentials - Summary.mp4
01:13
More details
User Reviews
Rating
average 0
Focused display
Category
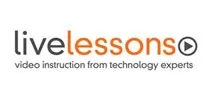
LiveLessons
View courses LiveLessonsPearson's video training library is an indispensable learning tool for today's competitive job market. Having essential technology training and certifications can open doors for career advancement and life enrichment. We take learning personally. We've published hundreds of up-to-date videos on wide variety of key topics for Professionals and IT Certification candidates. Now you can learn from renowned industry experts from anywhere in the world, without leaving home.
- language english
- Training sessions 109
- duration 10:35:57
- Release Date 2023/11/03