Introduction to Collections, Generics & Reflection in Java
Holczer Balazs
7:43:46
Description
A Guide to Understand Generics, Collections Framework (Data Structures), Stream API and Reflection in Java!
What You'll Learn?
- Understand the basics of generics
- Understand bounded type parameters
- Understand wildcards (unbounded, upper bounded and lower bounded wildcards)
- Understand type erasure and type inference
- Understand the basic data structures
- Understand hash maps and sets
- Understand lists (ArrayLists and LinkedLists)
- Understand stacks and queues
- Understand the Collection Framework
- Understand hashing and hash-functions
- Understand Stream API
Who is this for?
What You Need to Know?
More details
DescriptionLearn the basic concepts and functions that you will need to build fully functional programs with the popular programming language, Java.
This course is about generics in the main. You will lern the basics of generic types, generic methods, type parameters and the theoretical background concerning these topics. This is a fundamental part of Java so it is definitly worth learning.
Section 1 - Generic and Generic Programming
why to use generics and generic programming
Section 2 - Basic Generics
generic types
generic methods
fundamentals of generics and generic programming
Section 3 - Bounded Type Parameters
bounded type parameters
Section 4 - Type Inference
what is type inference
Section 5 - Wildcards
what are wildcards?
lower bounded wildcards
upper bounded wildcards
wildcards and bounded type parameters
Section 6 - Type Erasure
what is type erasure?
bridge methods and type erasure
Section 7 - Collections Framework
the collections framework
measuring the running time of algorithms
what are data structures?
Section 8 - Lists
ArrayList and LinkedList
performance comparison of LinkedLists and ArrayLists
Vectors
Stacks
Section 9 - Queues
what are queues?
PriorityQueues
ArrayDeques
Section 10 - Maps
hashing based data structures and hash-functions
HashMaps and LinkedHashMaps
balanced binary search trees and red-black trees
TreeMaps
Section 11 - Sets
what are sets?
HashSets, LinkedHashSets and TreeSets
Section 12 - Sorting Collections
sorting arrays and collections
Comparable and Comparator interfaces
sorting with lambda expressions
Section 13 - Stream API
streams
sequential streams and parallel streams
map() and flatMap()
reduce()
Section 13 - Reflection
what is reflection?
annotations and reflection
reflection and frameworks (such as Spring)
Learning the fundamentals of Java is a good choice and puts a powerful and tool at your fingertips. Java is easy to learn as well as it has excellent documentation, and is the base for all object-oriented programming languages.
Jobs in Java development are plentiful, and being able to learn Java will give you a strong background to pick up other object-oriented languages such as C++, or C# more easily.
Who this course is for:
- Beginner Java developers curious about generics, collections and reflection
Learn the basic concepts and functions that you will need to build fully functional programs with the popular programming language, Java.
This course is about generics in the main. You will lern the basics of generic types, generic methods, type parameters and the theoretical background concerning these topics. This is a fundamental part of Java so it is definitly worth learning.
Section 1 - Generic and Generic Programming
why to use generics and generic programming
Section 2 - Basic Generics
generic types
generic methods
fundamentals of generics and generic programming
Section 3 - Bounded Type Parameters
bounded type parameters
Section 4 - Type Inference
what is type inference
Section 5 - Wildcards
what are wildcards?
lower bounded wildcards
upper bounded wildcards
wildcards and bounded type parameters
Section 6 - Type Erasure
what is type erasure?
bridge methods and type erasure
Section 7 - Collections Framework
the collections framework
measuring the running time of algorithms
what are data structures?
Section 8 - Lists
ArrayList and LinkedList
performance comparison of LinkedLists and ArrayLists
Vectors
Stacks
Section 9 - Queues
what are queues?
PriorityQueues
ArrayDeques
Section 10 - Maps
hashing based data structures and hash-functions
HashMaps and LinkedHashMaps
balanced binary search trees and red-black trees
TreeMaps
Section 11 - Sets
what are sets?
HashSets, LinkedHashSets and TreeSets
Section 12 - Sorting Collections
sorting arrays and collections
Comparable and Comparator interfaces
sorting with lambda expressions
Section 13 - Stream API
streams
sequential streams and parallel streams
map() and flatMap()
reduce()
Section 13 - Reflection
what is reflection?
annotations and reflection
reflection and frameworks (such as Spring)
Learning the fundamentals of Java is a good choice and puts a powerful and tool at your fingertips. Java is easy to learn as well as it has excellent documentation, and is the base for all object-oriented programming languages.
Jobs in Java development are plentiful, and being able to learn Java will give you a strong background to pick up other object-oriented languages such as C++, or C# more easily.
Who this course is for:
- Beginner Java developers curious about generics, collections and reflection
User Reviews
Rating
Holczer Balazs
Instructor's Courses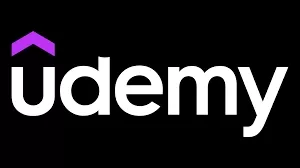
Udemy
View courses Udemy- language english
- Training sessions 76
- duration 7:43:46
- English subtitles has
- Release Date 2024/03/12