Go: The Complete Developer's Guide (Golang)
Stephen Grider
8:20:29
Description
Master the fundamentals and advanced features of the Go Programming Language (Golang)
What You'll Learn?
- Build massively concurrent programs with Go Routines and Channels
- Learn the advanced features of Go
- Understand the differences between commonly used data structures
- Prove your knowledge with dozens of included quiz questions
- Apply Interfaces to dramatically simplify complex programs
- Use types to future-proof your code and reduce the difficulty of refactors
Who is this for?
More details
DescriptionGo is an open source programming language created by Google. Â As one of the fastest growing languages in terms of popularity, its a great time to pick up the basics of Go!
This course is designed to get you up and running as fast as possible with Go.  We'll quickly cover the basics, then dive into some of the more advanced features of the language.  Don't be tricked by other courses that only teach you for-loops and if-statements!  This is the only course on Udemy that will teach you how to use the full power of Go's concurrency model and interface type systems.
Go is designed to be easy to pick up, but tough to master. Â Through multiple projects, quizzes, and assignments, you'll quickly start to master the language's quirks and oddities. Â Go is like any other language - you have to write code to learn it! Â This course will give you ample opportunities to strike out on your own and start working on your own programs.
In this course you will:
- Understand the basic syntax and control structures of the language
- Apply Go's concurrency model to build massively parallel systems
- Grasp the purpose of types, which is especially important if you're coming from a dynamically typed language like Javascript or Ruby
- Organize code through the use of packages
- Use the Go runtime to build and compile projects
- Get insight into critical design decisions in the language
- Gain a sense of when to use basic language features
Go is one of the fastest-growing programming languages released in the last ten years.  Get job-ready with Go today by enrolling now!
Who this course is for:
- Anyone who wants to understand the fundamental features of Go
Go is an open source programming language created by Google. Â As one of the fastest growing languages in terms of popularity, its a great time to pick up the basics of Go!
This course is designed to get you up and running as fast as possible with Go.  We'll quickly cover the basics, then dive into some of the more advanced features of the language.  Don't be tricked by other courses that only teach you for-loops and if-statements!  This is the only course on Udemy that will teach you how to use the full power of Go's concurrency model and interface type systems.
Go is designed to be easy to pick up, but tough to master. Â Through multiple projects, quizzes, and assignments, you'll quickly start to master the language's quirks and oddities. Â Go is like any other language - you have to write code to learn it! Â This course will give you ample opportunities to strike out on your own and start working on your own programs.
In this course you will:
- Understand the basic syntax and control structures of the language
- Apply Go's concurrency model to build massively parallel systems
- Grasp the purpose of types, which is especially important if you're coming from a dynamically typed language like Javascript or Ruby
- Organize code through the use of packages
- Use the Go runtime to build and compile projects
- Get insight into critical design decisions in the language
- Gain a sense of when to use basic language features
Go is one of the fastest-growing programming languages released in the last ten years.  Get job-ready with Go today by enrolling now!
Who this course is for:
- Anyone who wants to understand the fundamental features of Go
User Reviews
Rating
Stephen Grider
Instructor's Courses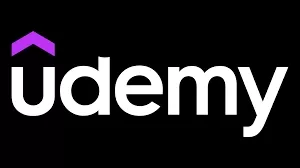
Udemy
View courses Udemy- language english
- Training sessions 78
- duration 8:20:29
- Release Date 2022/12/06