Getting Started with the C Language
Focused View
Giovanni Dicanio
2:18:50
58 View
01. Course Overview.mp4
02:05
02. Introduction.mp4
01:17
03. Some Important Applications of C.mp4
02:40
04. Starting with a Minimal Skeleton Code.mp4
02:58
06. The Role of the C Compiler.mp4
02:34
07. Compiling from the Command Line.mp4
02:20
08. Prerequisites for Setting Up VS Code for C Development on Windows.mp4
02:32
09. Adding a Source Code File to VS Code.mp4
00:59
10. Building Your C Code with VS Code.mp4
02:21
11. Setting Up the C C++ Debugger with VS Code.mp4
02:09
12. Debugging Your Code.mp4
01:33
13. Fixing a Build Error.mp4
01:59
14. An Exercise for the Learner.mp4
00:59
15. Summary.mp4
01:16
16. Introduction.mp4
01:07
17. Starting with a Concrete Example-Temperature Converter.mp4
01:48
18. Creating Variables.mp4
02:15
19. Naming Variables.mp4
01:34
20. Reading User Input with scanf.mp4
02:55
21. Processing Data with Operators.mp4
01:30
22. Printing Formatted Output with printf.mp4
01:48
23. Demo-Temperature Conversion in Action.mp4
02:46
24. Representing Integers with the int Type.mp4
01:14
25. Summary.mp4
01:10
26. Introduction.mp4
01:05
27. Creating String Variables in C.mp4
02:25
28. Memory Layout of C Strings.mp4
02:47
29. The char Type and Escape Sequences.mp4
02:35
30. Basic I O with Strings.mp4
03:12
31. Demo-Basic String I O in Action.mp4
02:10
32. Basic String Operations-String Copy and Concatenation.mp4
02:27
33. Writing Secure Code with Safe String Functions.mp4
02:32
34. Demo-String Manipulation Functions in Action.mp4
01:59
35. String Length vs. Size.mp4
01:11
36. Demo-String Length vs. Size in Action.mp4
01:03
37. A Brief Touch on Representing International Text with UTF-8.mp4
02:15
38. Summary.mp4
01:46
39. Introduction.mp4
00:45
40. Making Decisions with the if-else Statement.mp4
02:47
41. Introducing Relational and Logical Operators.mp4
01:35
42. Demo-if-else in Action.mp4
01:43
43. Making More Complex Decisions with the if-else-if Ladder.mp4
00:59
44. Demo-The if-else-if Ladder in Action.mp4
00:49
45. Demo-A Subtle Beginner Bug Involving the if Statement.mp4
02:45
46. Declaring Variables Inside if Statements.mp4
01:28
47. Nesting if Statements.mp4
00:41
48. Selecting between Multiple Values with the switch Statement.mp4
03:23
49. Demo-The switch Statement in Action.mp4
01:10
50. Simplifying Conditional Code with the Ternary Operator.mp4
01:32
51. Summary.mp4
00:56
52. Introduction.mp4
01:01
53. Iterating with the for Loop.mp4
03:23
54. Demo-The for Loop in Action.mp4
00:46
55. Demo-Underlining a String Using the for Loop.mp4
01:09
56. Demo-Printing a Multiplication Table with Nested for Loops.mp4
01:21
57. Iterating While a Condition Is True with while and do-while Loops.mp4
01:36
58. Demo-The while Loop in Action.mp4
02:09
59. Demo-do-while Loop in action.mp4
01:17
60. Changing the Normal Execution Flow with Control Statements.mp4
02:14
62. Summary.mp4
01:23
63. Introduction.mp4
01:17
64. Why Do You Need Functions.mp4
02:00
65. Writing Your First Function in C.mp4
04:58
66. Demo-Your First Function in Action.mp4
02:30
67. A Fresh New Look at main().mp4
01:33
68. Demo-A Swap Function with a Subtle Bug.mp4
05:08
69. Analyzing the Subtle Bug in the Swap Function.mp4
03:01
70. A Brief Introduction to Pointers.mp4
02:21
71. Parameter Passing by Reference Using Pointers.mp4
01:17
72. Demo-Fixing the Swap Function with Pointer Parameters.mp4
00:58
73. A Brief Touch on Other Applications of Pointers.mp4
01:37
74. Summary and Thank You.mp4
02:02
Description
This course will teach you in a practical way, with a combination of slides and demo code, the basic aspects of the C programming language and tools, so that you can be productive with the C language, and be able to write beginning applications in C.
What You'll Learn?
In this course you will learn the basic aspects of the C language programming and tools you will need to be productive with the C language.
More details
User Reviews
Rating
average 0
Focused display
Category
Giovanni Dicanio
Instructor's CoursesGiovanni Dicanio is a computer programmer specialized in both cross-platform C and C++, and Windows operating system development. Giovanni wrote computer programming articles on C++, OpenGL and other programming subjects on Italian computer magazines. He recently authored some C++ feature articles for MSDN Magazine, too. He contributed code to some open-source projects as well. His computer programming experience dates back to the glorious Commodore 64 and Amiga 500 golden days, with Basic and assembly. Giovanni likes helping people solving C and C++ programming problems on forums including Stack Overflow. He has held the Microsoft MVP Award for Visual C++ since 2007 for his contributions to the C++ development community, first on NNTP newsgroups then on forums.
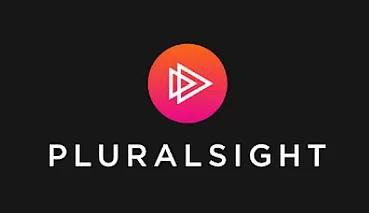
Pluralsight
View courses PluralsightPluralsight, LLC is an American privately held online education company that offers a variety of video training courses for software developers, IT administrators, and creative professionals through its website. Founded in 2004 by Aaron Skonnard, Keith Brown, Fritz Onion, and Bill Williams, the company has its headquarters in Farmington, Utah. As of July 2018, it uses more than 1,400 subject-matter experts as authors, and offers more than 7,000 courses in its catalog. Since first moving its courses online in 2007, the company has expanded, developing a full enterprise platform, and adding skills assessment modules.
- language english
- Training sessions 72
- duration 2:18:50
- level preliminary
- Release Date 2023/12/11