Getting started with Modern C#: Mastering the Basics
Nitish Kumar
6:24:34
Description
Learn C# (C Sharp) programming language | A complete and step by step course for beginners
What You'll Learn?
- Understand the basics of C# programming, including variables, data types, operators, and control structures.
- Set up a development environment and write the first C# program.
- Learn about the Console Class and its basic functionalities.
- Differentiate between Console .Write() and Console .WriteLine() methods.
- Understand the difference between Console .Read() and Console .ReadKey() methods.
- Customize the Console window by changing its color, height, and width.
- Explore various data types in C#, such as booleans, integers, floating-point numbers, and characters.
- Familiarize yourself with newly added data types like IntPtr and UIntPtr.
- Gain knowledge of string manipulation and perform operations using built-in methods.
- Understand the concepts of string immutability and mutability in C#.
- Learn the importance of the StringBuilder Class and its practical usage.
- Master string formatting techniques using String .Concat(), String .Format(), and string interpolation.
- Gain knowledge about the Object Class type and Var vs Dynamic keyword.
- Learn casting and type conversions, including implicit and explicit conversions.
- Understand the differences between Convert Class and Parse Methods.
- Write better code using the TryParse method and learn about boxing and unboxing.
- Manipulate and perform operations on various data types using C# operators.
- Practice arithmetic, comparison, equality, and logical operators.
- Explore the Math Class and utilize its built-in methods for performing operations.
- Develop a solid foundation in C# fundamentals for confidently building applications and pursuing advanced topics in C# development.
Who is this for?
What You Need to Know?
More details
DescriptionThis comprehensive C# course offers an in-depth exploration of C# programming, ensuring a strong grasp of its core principles. It caters to both beginners with no prior programming experience and experienced developers seeking to refresh their skills. The course establishes a solid foundation in C# programming through theoretical explanations, practical examples, and hands-on exercises, enabling participants to write clean, efficient, and reliable code using C#.
Course Objectives:
Introduction to C#:
- Acquire a fundamental understanding of C# programming, encompassing variables, data types, operators, and control structures.
- Learn to set up a development environment and create initial C# programs.
Working with the Console Class:
- Gain proficiency in the Console Class basics.
- Differentiate between Console .Write() and Console .WriteLine() methods.
- Understand the dissimilarities between Console .Read() and Console .ReadKey() methods.
- Customize the Console window by altering its background and foreground colors.
- Personalize the Console window by adjusting its dimensions.
Working with C# Data Types:
- Explore the various data types in C#, including booleans, integers, and integral numeric types (sbyte, byte, short, ushort, int, uint, long, ulong), floating-point numbers (float, double, decimal), and characters.
- Familiarize yourself with the newly introduced native-sized integers, IntPtr and UIntPtr, in C#.
Working with Strings:
- Develop a solid understanding of the fundamental aspects of string data type in C#.
- Perform a wide range of operations on string data type using built-in methods like Length, SubString, Trim, Split, Replace, and Remove.
- Comprehend the concepts of string immutability and mutability in C#.
- Recognize the significance of the StringBuilder Class and learn its practical usage through examples.
String Formatting:
- Learn various methods of formatting strings, including String.Concat(), String.Format(), and string interpolation.
Learn the Parent of All Data Types in C#:
- Understand the Object Class type.
- Differentiate between Var and Dynamic keywords through practical examples.
Casting and Type Conversions (C# Programming Guide):
- Learn implicit and explicit ways of converting data types.
- Understand the differences between Convert Class and Parse Methods.
- Enhance code quality by utilizing the TryParse method.
- Familiarize yourself with Boxing and Unboxing concepts for C# interviews.
Manipulating and Performing Operations on Various Data Types (C# Operators):
- Gain expertise in arithmetic operators, encompassing unary and binary operations such as increment, decrement, addition, subtraction, multiplication, and division.
- Learn to compare data types using comparison operators such as less than (), greater than (>), less than or equal to (), and greater than or equal to (>=).
- Master equality operators, including equal equal (==) and not equal (!=).
- Practice logical operators in C#.
- Understand the distinctions between & and && as well as | and || operators.
- Learn the utilization of the ! operator.
Learn to Perform Various Operations Using the Math Class:
- Explore the extensive range of built-in methods in the Math Class.
And many more topics to be covered soon...
Upon completion of this course, participants will possess a comprehensive understanding of C# fundamentals, empowering them to confidently develop their own C# applications and delve into advanced topics in C# development.
Who this course is for:
- Understand the basics of C# programming, including variables, data types, operators, and control structures.
- Explore the different data types available in C#, including integers, floating-point numbers, strings, arrays, and collections.
- Master the usage of control structures like if-else statements, loops, switch statements, and conditional expressions.
- Learn how to handle exceptions and errors in C# programs effectively.
- Discover techniques for debugging and troubleshooting common programming issues.
- Want to be a successful Dot Net Developer
This comprehensive C# course offers an in-depth exploration of C# programming, ensuring a strong grasp of its core principles. It caters to both beginners with no prior programming experience and experienced developers seeking to refresh their skills. The course establishes a solid foundation in C# programming through theoretical explanations, practical examples, and hands-on exercises, enabling participants to write clean, efficient, and reliable code using C#.
Course Objectives:
Introduction to C#:
- Acquire a fundamental understanding of C# programming, encompassing variables, data types, operators, and control structures.
- Learn to set up a development environment and create initial C# programs.
Working with the Console Class:
- Gain proficiency in the Console Class basics.
- Differentiate between Console .Write() and Console .WriteLine() methods.
- Understand the dissimilarities between Console .Read() and Console .ReadKey() methods.
- Customize the Console window by altering its background and foreground colors.
- Personalize the Console window by adjusting its dimensions.
Working with C# Data Types:
- Explore the various data types in C#, including booleans, integers, and integral numeric types (sbyte, byte, short, ushort, int, uint, long, ulong), floating-point numbers (float, double, decimal), and characters.
- Familiarize yourself with the newly introduced native-sized integers, IntPtr and UIntPtr, in C#.
Working with Strings:
- Develop a solid understanding of the fundamental aspects of string data type in C#.
- Perform a wide range of operations on string data type using built-in methods like Length, SubString, Trim, Split, Replace, and Remove.
- Comprehend the concepts of string immutability and mutability in C#.
- Recognize the significance of the StringBuilder Class and learn its practical usage through examples.
String Formatting:
- Learn various methods of formatting strings, including String.Concat(), String.Format(), and string interpolation.
Learn the Parent of All Data Types in C#:
- Understand the Object Class type.
- Differentiate between Var and Dynamic keywords through practical examples.
Casting and Type Conversions (C# Programming Guide):
- Learn implicit and explicit ways of converting data types.
- Understand the differences between Convert Class and Parse Methods.
- Enhance code quality by utilizing the TryParse method.
- Familiarize yourself with Boxing and Unboxing concepts for C# interviews.
Manipulating and Performing Operations on Various Data Types (C# Operators):
- Gain expertise in arithmetic operators, encompassing unary and binary operations such as increment, decrement, addition, subtraction, multiplication, and division.
- Learn to compare data types using comparison operators such as less than (), greater than (>), less than or equal to (), and greater than or equal to (>=).
- Master equality operators, including equal equal (==) and not equal (!=).
- Practice logical operators in C#.
- Understand the distinctions between & and && as well as | and || operators.
- Learn the utilization of the ! operator.
Learn to Perform Various Operations Using the Math Class:
- Explore the extensive range of built-in methods in the Math Class.
And many more topics to be covered soon...
Upon completion of this course, participants will possess a comprehensive understanding of C# fundamentals, empowering them to confidently develop their own C# applications and delve into advanced topics in C# development.
Who this course is for:
- Understand the basics of C# programming, including variables, data types, operators, and control structures.
- Explore the different data types available in C#, including integers, floating-point numbers, strings, arrays, and collections.
- Master the usage of control structures like if-else statements, loops, switch statements, and conditional expressions.
- Learn how to handle exceptions and errors in C# programs effectively.
- Discover techniques for debugging and troubleshooting common programming issues.
- Want to be a successful Dot Net Developer
User Reviews
Rating
Nitish Kumar
Instructor's Courses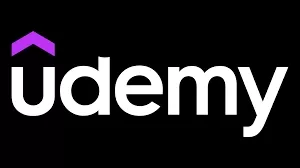
Udemy
View courses Udemy- language english
- Training sessions 34
- duration 6:24:34
- Release Date 2023/08/01