DSA in JS - Solving Basic Math Problems
Harish Krishnan
3:01:15
Description
Solve simple Math problems using JS
What You'll Learn?
- Basic Idea on Analysis of Algorithms
- Crash Course on Asymptotic Analysis
- Solve different Math problems using JS
- Practice using Coding Exercises
Who is this for?
What You Need to Know?
More details
DescriptionWelcome to this course DSA in JS - Solving Basic Math Problems.
This course is all about solving basic math problems using JavaScript. The course will follow a pattern to give learners an overview of the problem we are trying to solve visually and a coding exercise to solve the same. In the end, the solution is also provided as video explanations.
The course encompasses the below topics
Introduction to Analysis of Algorithms
Introduction to Asymptotic Analysis
Then we solve the below problems using JS
Counting Digits
Check Whether a Number is a Palindrome
Finding the Factorial of a Number
Finding the number of Trailing Zeroes
Finding Greatest Common Divisor (GCD)
Finding Least Common Multiple (LCM)
Finding whether a number is a Prime Number
Finding all Prime Factors
Finding Divisors of a Number
Sieve of Eratosthenes Algorithm
Computing the Powers of a number
Finding Absolute Value
Finding the Number of Digits in a Factorial
Finding the Exact Three Divisors
Modulo Addition
Module Multiplication
Throughout the course each problem is given an overview and a coding exercise to solve the same is provided, so you can try it out before looking at the solution videos. All codes are attached to the lectures for you to download as well.
Who this course is for:
- Beginner JavaScript Developers who likes to solve DSA Problems
Welcome to this course DSA in JS - Solving Basic Math Problems.
This course is all about solving basic math problems using JavaScript. The course will follow a pattern to give learners an overview of the problem we are trying to solve visually and a coding exercise to solve the same. In the end, the solution is also provided as video explanations.
The course encompasses the below topics
Introduction to Analysis of Algorithms
Introduction to Asymptotic Analysis
Then we solve the below problems using JS
Counting Digits
Check Whether a Number is a Palindrome
Finding the Factorial of a Number
Finding the number of Trailing Zeroes
Finding Greatest Common Divisor (GCD)
Finding Least Common Multiple (LCM)
Finding whether a number is a Prime Number
Finding all Prime Factors
Finding Divisors of a Number
Sieve of Eratosthenes Algorithm
Computing the Powers of a number
Finding Absolute Value
Finding the Number of Digits in a Factorial
Finding the Exact Three Divisors
Modulo Addition
Module Multiplication
Throughout the course each problem is given an overview and a coding exercise to solve the same is provided, so you can try it out before looking at the solution videos. All codes are attached to the lectures for you to download as well.
Who this course is for:
- Beginner JavaScript Developers who likes to solve DSA Problems
User Reviews
Rating
Harish Krishnan
Instructor's Courses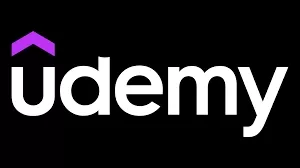
Udemy
View courses Udemy- language english
- Training sessions 51
- duration 3:01:15
- Release Date 2023/08/21