Develop an Interpreter Using TypeScript
Harish Krishnan
14:25:32
Description
Develop an interpreter from scratch without third-party libraries
What You'll Learn?
- Developing an Interpreter using TypeScript
- Understand Lexing, Parsing , Evaluation steps of the Parser
- Create a REPL for playing with the interpreter
- Develop interpreter for a custom programming language
- Develop and understand Pratt Parser logic
Who is this for?
What You Need to Know?
More details
DescriptionHello everyone welcome to the course Develop an Interpreter using TypeScript.
This course is a comprehensive and practical guide that takes you on a journey of building your own interpreter using the TypeScript language. This course is inspired by the great book Writing An Interpreter In Go by Thorsten Ball. All credit to him as well. We will demystify the complex world of interpreters in this course.
The course begins by introducing the fundamental concepts of interpreters and programming languages, making it accessible to both beginners and experienced developers. We will build an interpreter using TypeScript for a custom programming language called Monkey through a hands-on approach.
we will understand key concepts such as lexical analysis, parsing, and evaluating expressions. In the course we will learn how to design and implement a lexer and a recursive descent parser, providing you learners with a solid foundation in language processing techniques.
The course will help developers seeking to deepen their understanding of language implementation.
During the process of developing an interpreter, we will also learn more advanced topics like closures, first-class functions, and error handling.
By the end of the course, you would have hopefully gained a deep understanding of how interpreters work and the skills to build your own programming language, opening up new possibilities for exploration and creativity in the world of software development.
Who this course is for:
- Intermediate JavaScript / TypeScript developers
Hello everyone welcome to the course Develop an Interpreter using TypeScript.
This course is a comprehensive and practical guide that takes you on a journey of building your own interpreter using the TypeScript language. This course is inspired by the great book Writing An Interpreter In Go by Thorsten Ball. All credit to him as well. We will demystify the complex world of interpreters in this course.
The course begins by introducing the fundamental concepts of interpreters and programming languages, making it accessible to both beginners and experienced developers. We will build an interpreter using TypeScript for a custom programming language called Monkey through a hands-on approach.
we will understand key concepts such as lexical analysis, parsing, and evaluating expressions. In the course we will learn how to design and implement a lexer and a recursive descent parser, providing you learners with a solid foundation in language processing techniques.
The course will help developers seeking to deepen their understanding of language implementation.
During the process of developing an interpreter, we will also learn more advanced topics like closures, first-class functions, and error handling.
By the end of the course, you would have hopefully gained a deep understanding of how interpreters work and the skills to build your own programming language, opening up new possibilities for exploration and creativity in the world of software development.
Who this course is for:
- Intermediate JavaScript / TypeScript developers
User Reviews
Rating
Harish Krishnan
Instructor's Courses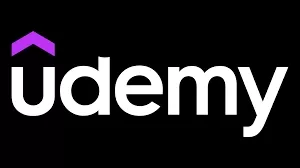
Udemy
View courses Udemy- language english
- Training sessions 96
- duration 14:25:32
- English subtitles has
- Release Date 2023/08/15