Design Patterns in Python 3
Gerald Britton
4:39:55
Description
This course will teach you how to use proven object-oriented design patterns to significantly enhance the stability, testability, and maintainability of your Python development while decreasing your development time.
What You'll Learn?
Whether you're an experienced Python developer or just getting started, having ready-to-use solutions in your toolkit will make your job easier and more satisfying. Thanks to the famous “Gang of Four,” there are 24 essential design patterns you can easily use in Python. In this course, Design Patterns in Python 3, you’ll learn to use object-oriented design patterns in Python. First, you’ll explore the origins of design patterns and their applicability to programming projects of all sizes. Next, you’ll discover the many design patterns described in the well-known “Gang of Four” book on the topic. Finally, you’ll learn how to apply these patterns to solve real-world problems that occur in businesses and organizations of all sizes. When you’re finished with this course, you’ll have the skills and knowledge of object-oriented Python programming needed to build stable, maintainable, and extensible applications.
More details
User Reviews
Rating
Gerald Britton
Instructor's Courses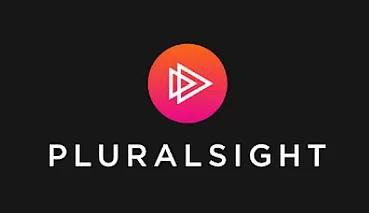
Pluralsight
View courses Pluralsight- language english
- Training sessions 154
- duration 4:39:55
- level advanced
- English subtitles has
- Release Date 2023/06/15