Design Patterns in Java - Beginner to Expert
Robert Gioia
3:45:12
Description
Go from zero knowledge of design patterns to knowing how to implement all 23 of them in Java!
What You'll Learn?
- Understand and implement all 23 Design Patterns
- Identify when to use creational, structural, and behavioral design patterns
- Master creational design patterns like Singleton, Builder, and Factory Method
- Implement structural design patterns like Adapter, Bridge, and Composite
- Be proficient in behavioral design patterns including Memento and Visitor
- Understand and implement searching and sorting algorithms in Java
- Know what a Binary Search is and how to implement it
- Master sorting algorithms like bubble sort, quick sort, and merge sort
Who is this for?
What You Need to Know?
More details
DescriptionThis course is designed to take a Java programmer with any level of experience beginner, intermediate, or advanced and provide them with a deep understanding of design patterns and algorithms.
In this course you will master the 23 Design Patterns in computer programming written about in the classic book Design Patterns: Elements of Object-Oriented Software. In this book, four incredible software developers - Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides - compile reusable solutions to commonly occurring problems when writing computer programs. These patterns are grouped into 3 categories:Â creational, structural, and behavioral. Creational design patterns are used to create objects. Structural design patterns are used to assemble objects from reusable components. Behavioral design patterns architect how objects in the program interact with each other.
This course is split into four main parts:
Creational Design Patterns
We'll learn how to create objects using clean code and reusable practices that make our code modular, scalable, and efficient. In this part of the course we will be learning how to implement the Abstract Factory, Builder, Factory Method, Prototype, and Singleton design patterns.
Structural Design Patterns
We'll learn how to assemble complex objects from simple ones with tried and true techniques. We'll be learning how to implement the Adapter, Bridge, Composite, Decorator, Facade, Flyweight, and Proxy design patterns.
Behavioral Design Patterns
We'll gain an understanding of the various strategies used to architect how objects in the program will interact as we learn about the Chain of Responsibility, Command, Interpreter, Iterator, Mediator, Memento, Observer, State, Strategy, Template Method, and Visitor design patterns.
Searching and Sorting Algorithms
This additional section to the course will cover the most popular searching and sorting algorithms in all of computer science. We will be learning about Linear Search, Binary Search, Selection Sort, Bubble Sort, Quick Sort, Merge Sort, Insertion Sort, and Radix Sort.
By the end of this course you will be proficient in the most popular and useful design patterns, searching algorithms, and sorting algorithms in the industry.
Who this course is for:
- Any level of Java developer that wants to learn Design Patterns & Algorithms using Java code
- Anyone that wants to learn all 23 design patterns from the Elements of Reusable Object-Oriented Software book
This course is designed to take a Java programmer with any level of experience beginner, intermediate, or advanced and provide them with a deep understanding of design patterns and algorithms.
In this course you will master the 23 Design Patterns in computer programming written about in the classic book Design Patterns: Elements of Object-Oriented Software. In this book, four incredible software developers - Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides - compile reusable solutions to commonly occurring problems when writing computer programs. These patterns are grouped into 3 categories:Â creational, structural, and behavioral. Creational design patterns are used to create objects. Structural design patterns are used to assemble objects from reusable components. Behavioral design patterns architect how objects in the program interact with each other.
This course is split into four main parts:
Creational Design Patterns
We'll learn how to create objects using clean code and reusable practices that make our code modular, scalable, and efficient. In this part of the course we will be learning how to implement the Abstract Factory, Builder, Factory Method, Prototype, and Singleton design patterns.
Structural Design Patterns
We'll learn how to assemble complex objects from simple ones with tried and true techniques. We'll be learning how to implement the Adapter, Bridge, Composite, Decorator, Facade, Flyweight, and Proxy design patterns.
Behavioral Design Patterns
We'll gain an understanding of the various strategies used to architect how objects in the program will interact as we learn about the Chain of Responsibility, Command, Interpreter, Iterator, Mediator, Memento, Observer, State, Strategy, Template Method, and Visitor design patterns.
Searching and Sorting Algorithms
This additional section to the course will cover the most popular searching and sorting algorithms in all of computer science. We will be learning about Linear Search, Binary Search, Selection Sort, Bubble Sort, Quick Sort, Merge Sort, Insertion Sort, and Radix Sort.
By the end of this course you will be proficient in the most popular and useful design patterns, searching algorithms, and sorting algorithms in the industry.
Who this course is for:
- Any level of Java developer that wants to learn Design Patterns & Algorithms using Java code
- Anyone that wants to learn all 23 design patterns from the Elements of Reusable Object-Oriented Software book
User Reviews
Rating
Robert Gioia
Instructor's Courses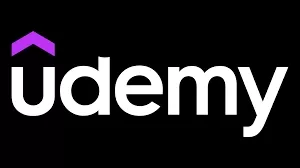
Udemy
View courses Udemy- language english
- Training sessions 49
- duration 3:45:12
- Release Date 2024/04/23