Data Structures for Absolute Beginners in Python
Abdul Rauf
6:42:42
Description
Understand and Implement data structures from absolute scratch in python. Learn about computational complexity.
What You'll Learn?
- Understand the most important data structures
- Implement Data structures from scratch in python
- Analyze algorithms using the big O notation
- Reverse a linked list
- Understand and implement stacks, queues, trees, linked lists and graphs from scratch
Who is this for?
More details
DescriptionYou'll learn about the most important and basic data structures that every veteran programmer knows about in detail. By the end of the course, you'll be able to implement these from absolute scratch.
The course is designed to help the students write their own code. Each module has explanation parts and implementation parts, you're encouraged to implement an explained topic on your own.
Data Structures covered in the course.
Singly Linked Lists
Doubly Linked Lists
Stacks
Queues
Binary Search Trees
Graphs
Computational Complexity
Algorithms
Reversing a linked list
Recursive and iterative tree traversal
Breadth First Search
Depth First Search
Analysis
Big O notation
Who is this course for?
Many people learn programming and are told to learn data structures and algorithms to pass coding interviews and get jobs. This course is designed for an individual who has some python programming knowledge and wants to get better at it by learning data structures. Even if you have no python experience but know some other programming language, this can be a great chance to learn python. For people who don't know python, this provides programming problems to get better at python.
How the course is designed
The course is designed with 7 major modules. We go from installing python, to setting up jupyter notebook to understanding and implementing data structures from scratch. We also visualize computational complexity of different operations we write for these data structures in the final section on computational complexity.
What will you get out of it
Better understanding of data structures.
More tools at your disposal for solving programming problems.
Ability to implement data structures from scratch in python.
Opportunity to practice python.
Who this course is for:
- Beginner python programmers curious about data structures
- Beginners wanting to up their programming game
- Beginners interested in learning about data structures from scratch
You'll learn about the most important and basic data structures that every veteran programmer knows about in detail. By the end of the course, you'll be able to implement these from absolute scratch.
The course is designed to help the students write their own code. Each module has explanation parts and implementation parts, you're encouraged to implement an explained topic on your own.
Data Structures covered in the course.
Singly Linked Lists
Doubly Linked Lists
Stacks
Queues
Binary Search Trees
Graphs
Computational Complexity
Algorithms
Reversing a linked list
Recursive and iterative tree traversal
Breadth First Search
Depth First Search
Analysis
Big O notation
Who is this course for?
Many people learn programming and are told to learn data structures and algorithms to pass coding interviews and get jobs. This course is designed for an individual who has some python programming knowledge and wants to get better at it by learning data structures. Even if you have no python experience but know some other programming language, this can be a great chance to learn python. For people who don't know python, this provides programming problems to get better at python.
How the course is designed
The course is designed with 7 major modules. We go from installing python, to setting up jupyter notebook to understanding and implementing data structures from scratch. We also visualize computational complexity of different operations we write for these data structures in the final section on computational complexity.
What will you get out of it
Better understanding of data structures.
More tools at your disposal for solving programming problems.
Ability to implement data structures from scratch in python.
Opportunity to practice python.
Who this course is for:
- Beginner python programmers curious about data structures
- Beginners wanting to up their programming game
- Beginners interested in learning about data structures from scratch
User Reviews
Rating
Abdul Rauf
Instructor's Courses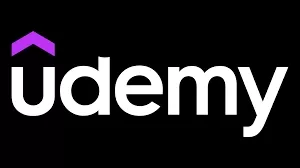
Udemy
View courses Udemy- language english
- Training sessions 63
- duration 6:42:42
- Release Date 2023/03/29