Data Structures and Algorithms in Python
Glassbyte Team
11:39:50
Description
Visualize the inner workings of data structures and algorithms, line by line, through comprehensive animations
What You'll Learn?
- Understand how computers store and work with data
- Analyze the space and memory utilization of any algorithm using the Big O Notation
- Dive deep into the mechanisms of function execution and demystify the concept of Recursion
- Implement from scratch the most common 9 Data Structures
- Understand how the best known 8 sorting algorithms work
- Learn the searching / traversing algorithms for trees and graphs
- Improve your problem solving skills
- Learn the behind the scenes of the basic data structures (arrays and hash maps)
- Understand common programming patterns
- Learn everything you need to know for the technical interview
Who is this for?
What You Need to Know?
More details
Description  Building reliable and highly performant software requires knowledge that goes beyond a certain programming language or framework. It requires a solid understanding of how data is organized in memory, how it can be manipulated, sorted or searched into. Thereâs a reason why all the big tech companies such as Google, Amazon or Netflix focus their technical interviews on those topics. Whether you do mobile apps, websites, games, machine learning or any other work that involves coding, you need a good grasp of Data Structures and Algorithms.
  Many self taught developers and aspiring engineers often feel they lack the knowledge when having to decide on the right data structure or the right approach for solving a problem. If you ever felt that way, this material is the right choice for you. This course packs months of Computer Science subject matter to get you on the same level of proficiency as someone with a Computer Science degree.
What sets us apart ?
  Every video begins with an in depth analysis of the topic at hand. At this stage we wonât write any code yet, but rather learn how to approach the problem, think of ways we could solve it and build a mental model of the solution.
  We then go on to code the algorithm step by step. But we donât stop there. We take one or more examples and walk through the code line by line. And we mean that literally. You will see how the code runs from top to bottom and how data flows and changes during execution. We find this method the absolute best way to really understand the inner workings of an algorithm.
  Weâll also analyze the time performance and space utilization of every algorithm and method we write using the Big O Notation. Weâll talk about the strengths and weaknesses of each data structure and discuss their real world usage. Apart from all of that, youâll also learn things like recursion, how computers work under the hood, problem solving techniques, common programming patterns and much more.
What does this course cover ?
How computers work under the hood
What a data structure is
What an algorithm is
Problem solving techniques
Big O Notation - how to analyze the time performance and space utilization of algorithms. This is done for every single function/algorithm we write.
Gain a deeper understanding of how code works
Recursion
Data Structures:
Arrays
Hash Tables
Singly Linked Lists
Doubly Linked Lists
Stacks
Queues
Binary Search Trees
Tree Traversal
Heaps
Graphs
Sorting Algorithms:
Insertion Sort
Selection Sort
Bubble Sort
Shell Sort
Heap Sort
Merge Sort
Quick Sort
Radix Sort
Thanks for considering, and I hope this course will help you in your journey. Happy learning!
Who this course is for:
- Self-taught engineers with a career in a different field that want to switch to the tech industry
- Anyone who is preparing for a technical interview
- Engineers who want to build better tech fluency, land better roles, and push their career to new heights
- Computer Science students who want to supplement their studies with alternative learning materials
  Building reliable and highly performant software requires knowledge that goes beyond a certain programming language or framework. It requires a solid understanding of how data is organized in memory, how it can be manipulated, sorted or searched into. Thereâs a reason why all the big tech companies such as Google, Amazon or Netflix focus their technical interviews on those topics. Whether you do mobile apps, websites, games, machine learning or any other work that involves coding, you need a good grasp of Data Structures and Algorithms.
  Many self taught developers and aspiring engineers often feel they lack the knowledge when having to decide on the right data structure or the right approach for solving a problem. If you ever felt that way, this material is the right choice for you. This course packs months of Computer Science subject matter to get you on the same level of proficiency as someone with a Computer Science degree.
What sets us apart ?
  Every video begins with an in depth analysis of the topic at hand. At this stage we wonât write any code yet, but rather learn how to approach the problem, think of ways we could solve it and build a mental model of the solution.
  We then go on to code the algorithm step by step. But we donât stop there. We take one or more examples and walk through the code line by line. And we mean that literally. You will see how the code runs from top to bottom and how data flows and changes during execution. We find this method the absolute best way to really understand the inner workings of an algorithm.
  Weâll also analyze the time performance and space utilization of every algorithm and method we write using the Big O Notation. Weâll talk about the strengths and weaknesses of each data structure and discuss their real world usage. Apart from all of that, youâll also learn things like recursion, how computers work under the hood, problem solving techniques, common programming patterns and much more.
What does this course cover ?
How computers work under the hood
What a data structure is
What an algorithm is
Problem solving techniques
Big O Notation - how to analyze the time performance and space utilization of algorithms. This is done for every single function/algorithm we write.
Gain a deeper understanding of how code works
Recursion
Data Structures:
Arrays
Hash Tables
Singly Linked Lists
Doubly Linked Lists
Stacks
Queues
Binary Search Trees
Tree Traversal
Heaps
Graphs
Sorting Algorithms:
Insertion Sort
Selection Sort
Bubble Sort
Shell Sort
Heap Sort
Merge Sort
Quick Sort
Radix Sort
Thanks for considering, and I hope this course will help you in your journey. Happy learning!
Who this course is for:
- Self-taught engineers with a career in a different field that want to switch to the tech industry
- Anyone who is preparing for a technical interview
- Engineers who want to build better tech fluency, land better roles, and push their career to new heights
- Computer Science students who want to supplement their studies with alternative learning materials
User Reviews
Rating
Glassbyte Team
Instructor's Courses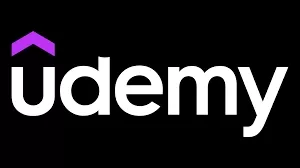
Udemy
View courses Udemy- language english
- Training sessions 79
- duration 11:39:50
- Release Date 2023/10/12