Data Structure & Algorithms Complete Course in Java
Ravi Singh
20:53:54
Description
Master Data Structure & Algorithms Course For Cracking Coding Interviews for Top product-based Companies.
What You'll Learn?
- Data Structure and Algorithms
- Coding Interview Questions
- Problem solving skill
- Crack product based company interviews
Who is this for?
More details
DescriptionData structure, Algorithms Course For Cracking coding for Top product-based companies. Each topic explains from very basic to an advanced level by using multiple examples. More focus is on Tricks, Techniques, and implementation than theory.
This course explains all the deep concepts of Data structure and Algorithms with the help of problems. These problems are frequently asked during interviews.
This course is for students in colleges preparing for campus placements and also for working professionals who want to crack interviews.
Course Prerequisite:Â Should have basic knowledge of Java or Python or C/C++ Programming
Who Should Subscribe For this Course:
⢠Working professionals preparing for interviews of Big-5(Google,Amazon,Microsoft,Facebook,Apple) and Top
Product Based organization
⢠BTech /MCA/MS Students looking for data structures, Algorithms & System Design coaching
⢠Job seekers who are preparing for interviews in Product MNC/e-commerce companies
⢠Anyone who has a deep desire to learn data structures, algorithms, and system design to improve
programming/coding skills and designing skills
Our instructor [ Ravi Singh] is a working professional who graduated from premier institutes and works with premier
companies Like: Amazon, Cisco, Walmart Labs
HOW IT WORKS
Finish Topics of the course:Â This course is divided into multiple topics. Subscribers can finish topics one after another. Attempt each topic assignment.
Who this course is for:
- Candidates curious for Data Structure & Algorithms interview preparation
- Prepare for Coding Interview
- Switch from service based company to product based company
- Want to increase salary by 2X ,3X, 4X, 5X
- Our student cracked interview with Microsoft with max package 1.2cr
Data structure, Algorithms Course For Cracking coding for Top product-based companies. Each topic explains from very basic to an advanced level by using multiple examples. More focus is on Tricks, Techniques, and implementation than theory.
This course explains all the deep concepts of Data structure and Algorithms with the help of problems. These problems are frequently asked during interviews.
This course is for students in colleges preparing for campus placements and also for working professionals who want to crack interviews.
Course Prerequisite:Â Should have basic knowledge of Java or Python or C/C++ Programming
Who Should Subscribe For this Course:
⢠Working professionals preparing for interviews of Big-5(Google,Amazon,Microsoft,Facebook,Apple) and Top
Product Based organization
⢠BTech /MCA/MS Students looking for data structures, Algorithms & System Design coaching
⢠Job seekers who are preparing for interviews in Product MNC/e-commerce companies
⢠Anyone who has a deep desire to learn data structures, algorithms, and system design to improve
programming/coding skills and designing skills
Our instructor [ Ravi Singh] is a working professional who graduated from premier institutes and works with premier
companies Like: Amazon, Cisco, Walmart Labs
HOW IT WORKS
Finish Topics of the course:Â This course is divided into multiple topics. Subscribers can finish topics one after another. Attempt each topic assignment.
Who this course is for:
- Candidates curious for Data Structure & Algorithms interview preparation
- Prepare for Coding Interview
- Switch from service based company to product based company
- Want to increase salary by 2X ,3X, 4X, 5X
- Our student cracked interview with Microsoft with max package 1.2cr
User Reviews
Rating
Ravi Singh
Instructor's Courses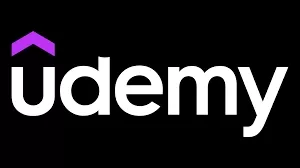
Udemy
View courses Udemy- language english
- Training sessions 94
- duration 20:53:54
- Release Date 2023/03/07