C# Object-Oriented Programming Fundamentals
Paul D. Sheriff
2:11:18
Description
Learn the skills you need to become proficient at C# object-oriented programming
What You'll Learn?
- Use Properties and Methods for Proper Class Design
- Use inheritance to cut down the amount of code you need to write
- Learn to control the visibility and the lifetime of data
- Raise and consume events from your classes
- Eliminate multiple methods by using a generic method
- Learn to manipulate generic collections
- Create your own class libraries for a proper N-Tier application
Who is this for?
More details
DescriptionWhen programming C#, or any .NET language for that matter, Object-Oriented Programming (OOP) is a required skill. This course provides you with the skills you need to master OOP in C#. In this course you are given a high-level overview of OOP terms before you drill down into each concept.
Creating classes with properties and methods is the first step in understanding OOP. Next, you learn how to use inheritance effectively to reduce the amount of code you write. Best practices around the scope of variables, classes, properties, and methods are also illustrated with several demos. Creating your own generic methods and classes will also greatly reduce the amount of code you need to write. Using the built-in .NET generic collections makes working with sets of data a breeze. Finally, you learn how to create your own class libraries.
All the demos you are shown during this course are backed up with a set of labs for you to perform. Walking through these labs step-by-step ensures that you understand the concepts illustrated in each lesson. You are going to see over 40 demos, be asked over 30 questions, and perform over 30 hands-on labs.
Join Paul D. Sheriff, one of the best instructors in the industry, on your journey to learning to an OOP C# developer.
Who this course is for:
- Any developer wishing to develop in the .NET framework with C# should take this course.
- If you are going to develop Web, Cloud, Mobile, Games, Internet of Things (IoT), or Desktop applications, OOP is a required skill.
- Any manager or QA person who needs to communicate better with their programming team should also take this course.
When programming C#, or any .NET language for that matter, Object-Oriented Programming (OOP) is a required skill. This course provides you with the skills you need to master OOP in C#. In this course you are given a high-level overview of OOP terms before you drill down into each concept.
Creating classes with properties and methods is the first step in understanding OOP. Next, you learn how to use inheritance effectively to reduce the amount of code you write. Best practices around the scope of variables, classes, properties, and methods are also illustrated with several demos. Creating your own generic methods and classes will also greatly reduce the amount of code you need to write. Using the built-in .NET generic collections makes working with sets of data a breeze. Finally, you learn how to create your own class libraries.
All the demos you are shown during this course are backed up with a set of labs for you to perform. Walking through these labs step-by-step ensures that you understand the concepts illustrated in each lesson. You are going to see over 40 demos, be asked over 30 questions, and perform over 30 hands-on labs.
Join Paul D. Sheriff, one of the best instructors in the industry, on your journey to learning to an OOP C# developer.
Who this course is for:
- Any developer wishing to develop in the .NET framework with C# should take this course.
- If you are going to develop Web, Cloud, Mobile, Games, Internet of Things (IoT), or Desktop applications, OOP is a required skill.
- Any manager or QA person who needs to communicate better with their programming team should also take this course.
User Reviews
Rating
Paul D. Sheriff
Instructor's Courses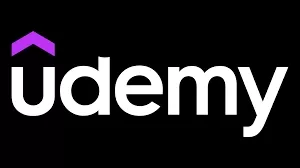
Udemy
View courses Udemy- language english
- Training sessions 65
- duration 2:11:18
- Release Date 2023/02/06