C++ Game Engine Programming
Focused View
31:46:10
0 View
001. Motivations & Learning Outcomes.mp4
16:28
002. How to Take this Course.mp4
02:58
003. Project Technologies & Libraries.mp4
22:05
004. Linux Dependencies.mp4
06:23
005. macOS Dependencies.mp4
02:35
006. A Small Note for Windows Programmers.mp4
03:39
007. Compilation.mp4
11:10
008. Header & Implementation Files.mp4
06:13
009. Linking.mp4
11:38
010. Makefile.mp4
08:35
011. Lua Version & Lua Header Files.mp4
04:47
012. Compiling & Testing All Dependencies.mp4
15:42
013. Configuring Visual Studio on Windows.mp4
34:21
014. Library Binaries.mp4
09:32
015. Game Loop.mp4
08:48
016. Game Class.mp4
18:05
017. Creating an SDL Window.mp4
15:45
018. Polling SDL Events.mp4
13:40
019. Rendering our SDL Window.mp4
06:50
020. Fullscreen Window.mp4
08:30
021. Fake Fullscreen vs. Real Fullscreen.mp4
03:53
022. Drawing an SDL Rectangle.mp4
06:40
023. Double-Buffered Renderer.mp4
02:32
024. Loading PNG Textures.mp4
10:14
025. Object Movement & Velocity Vectors.mp4
11:05
026. Capping the Game Framerate.mp4
15:19
027. SDL Delay.mp4
07:38
028. Delta Time.mp4
13:28
029. Uncapped Framerate.mp4
01:43
030. Logger Class.mp4
12:24
031. Logger Class Implementation.mp4
18:32
032. Popular Logging Libraries.mp4
04:40
033. Source Subfolders.mp4
05:12
034. Makefile Variables.mp4
12:26
035. Creating C++ Objects.mp4
15:23
036. Examples of C++ Object Creation.mp4
05:36
037. Organizing Game Objects.mp4
07:50
038. Object Inheritance Design.mp4
05:18
039. Component-Based Design.mp4
16:26
040. Entity-Component-System Design.mp4
22:09
041. ECS Folder Structure.mp4
21:02
042. System Component Signature.mp4
17:26
043. Working with C++ Templates.mp4
07:58
044. Component Type Template.mp4
10:41
045. Exercise System Functions.mp4
06:15
046. Adding & Removing Entities from Systems.mp4
08:13
047. Operator Overloading for Entities.mp4
10:40
048. Component Pool.mp4
11:30
049. The Pool Class.mp4
07:17
050. Implementing the Pool Class.mp4
13:45
051. Registry Systems & Entity Signatures.mp4
10:54
052. Entity Creation & Management.mp4
17:54
053. Function Templates to Manage Components.mp4
11:59
054. Adding Components.mp4
17:54
055. Function to Add Components.mp4
18:23
056. Function to Remove Components.mp4
07:10
057. Templates as Placeholders.mp4
07:54
058. Implementing System Functions.mp4
29:40
059. Creating our First Entity.mp4
11:21
060. Smart Pointers.mp4
14:57
061. Converting ECS Code to Smart Pointers.mp4
11:44
062. SDL Raw Pointers.mp4
03:33
063. Adding our First Components.mp4
10:05
064. Exercise Entity Class Managing Components.mp4
17:49
065. Entity Class Managing Components.mp4
07:25
066. A Warning About Cyclic Dependenciesb.mp4
02:19
067. Movement System.mp4
16:02
068. Movement System & Delta Time.mp4
03:29
069. Render System.mp4
17:27
070. Managing Game Assets.mp4
13:55
071. The Asset Store.mp4
23:41
072. Displaying Textures in our Render System.mp4
20:28
073. Exercise Displaying the Tilemap.mp4
08:18
074. Displaying the Tilemap.mp4
15:06
075. Rendering Order.mp4
17:48
076. Sorting Sprites by Z-Index.mp4
16:21
077. Animated Sprites.mp4
06:34
078. Animation System.mp4
27:42
079. Entity Collision Check.mp4
18:14
080. Implementing the Collision System.mp4
27:55
081. Exercise Render Collider Rectangle.mp4
02:21
082. Render Collider Rectangle.mp4
06:08
083. Killing Entities & Re-Using IDs.mp4
09:59
084. Implementing Entity Removal.mp4
20:00
085. Introduction to Event Systems.mp4
11:26
086. Event System Design Options.mp4
12:40
087. Starting to Code the Event System.mp4
10:50
088. Event Handlers.mp4
30:37
089. Emitting Events & Subscribing to Events.mp4
19:23
090. Exercise Key Pressed Event.mp4
03:31
091. Implementing the Key Pressed Event.mp4
07:32
092. Event System Design Patterns.mp4
06:27
093. Keyboard Control System.mp4
21:17
094. Camera Follow System.mp4
23:59
095. Sprites with Fixed Position.mp4
06:08
096. Camera Movement for Colliders.mp4
03:04
097. Projectile Emitter Component.mp4
05:20
098. Health Component.mp4
32:03
099. Projectile Duration.mp4
14:21
100. Exercise Shooting Projectiles.mp4
03:06
101. Shooting Projectiles.mp4
13:27
102. Tags & Groups.mp4
11:19
103. Optimizing Access of Tags & Groups.mp4
05:48
104. Implementing Tags & Groups.mp4
22:49
105. Projectiles Colliding with Player.mp4
18:58
106. Projectiles Colliding with Enemies.mp4
04:29
107. Error Checking and Validation.mp4
05:20
108. Data-Oriented Design.mp4
18:20
109. Avoiding Data Gaps.mp4
07:29
110. Packed Pool of Components.mp4
20:58
111. Coding Packed Pools of Data.mp4
31:03
112. Checking for Null Pool.mp4
01:54
113. Array of Structs vs. Struct of Arrays.mp4
14:21
114. Cache Profiling with Valgrind.mp4
10:17
115. Popular ECS Libraries.mp4
15:29
116. Adding Fonts to the Asset Store.mp4
13:04
117. Render Text System.mp4
27:00
118. Exercise Display Health Values.mp4
03:11
119. Rendering Health Values.mp4
20:25
120. Introduction to Dear ImGui.mp4
18:21
121. Dear ImGui Demo Window.mp4
24:18
122. Immediate-Mode GUI Paradigm.mp4
19:40
123. Render GUI System.mp4
17:58
124. Button to Spawn Enemies.mp4
15:18
125. Exercise Customizing New Enemy.mp4
07:14
126. Custom Enemy Values with ImGui.mp4
33:36
127. Killing Entities Outside Map Limits.mp4
08:26
128. Flipping Sprites on Collision.mp4
17:25
129. Exercise Keep Player Inside the Map.mp4
01:44
130. Keeping the Player Inside the Map.mp4
03:37
131. Culling Sprites Outside Camera View.mp4
10:40
132. Do Not Cull Fixed Sprites.mp4
01:42
133. Game Scripting.mp4
15:11
134. The Lua Scripting Language.mp4
08:40
135. Lua State.mp4
22:44
136. Reading Lua Tables.mp4
13:08
137. Lua Functions.mp4
16:33
138. Level Loader Class.mp4
19:44
139. Reading Assets from a Lua Table.mp4
27:12
140. Reading Entities from a Lua Table.mp4
21:21
141. Handling Multiple Objects in a Lua Level.mp4
08:32
142. Scripting Night-Day Tilemap.mp4
12:37
143. Scripting Entity Behavior with Lua.mp4
09:54
144. Script System.mp4
13:58
145. Lua Bindings.mp4
18:22
146. Binding Multiple Lua Functions.mp4
12:15
147. Loading Different Lua Levels.mp4
03:53
148. Division of C++ & Lua Code.mp4
04:49
149. Next Steps.mp4
06:25
More details
User Reviews
Rating
average 0
Focused display
Category
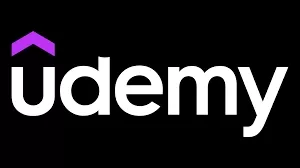
Udemy
View courses UdemyStudents take courses primarily to improve job-related skills.Some courses generate credit toward technical certification. Udemy has made a special effort to attract corporate trainers seeking to create coursework for employees of their company.
- language english
- Training sessions 149
- duration 31:46:10
- Release Date 2024/11/03