Advanced C# 7 Collections
Simon Robinson
3:46:05
Description
Learn to use the full range of Microsoft collections, from lists and dictionaries to sets, queues, and concurrent and immutable collections. This course will explore the principles of ensuring code with collections is scalable and robust.
What You'll Learn?
Understanding how to use collections properly is a vital part in writing effective C# applications. In this course, Advanced C# Collections, you'll learn how to use the full range of Microsoft collections and understand how to ensure collection elements are unique using sets, along with customizing dictionary key lookup and sorting of elements. First, you’ll develop an understanding of the principles of collection scalability, which is important to ensure performance stays acceptable when dealing with very large collections. Then, you'll discover how to make your code around collections more robust by using collection interfaces to decouple code, and read-only and immutable collections to protect your data. Finally, you'll delve into using collections in a multi-threaded scenario using the concurrent collections. By the end of this course, you'll have the knowledge needed to effectively choose the most appropriate collection for any reasonable situation, and leverage that collection following good practices.
More details
User Reviews
Rating
Simon Robinson
Instructor's Courses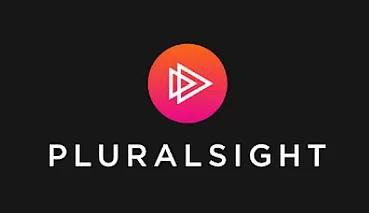
Pluralsight
View courses Pluralsight- language english
- Training sessions 97
- duration 3:46:05
- level average
- Release Date 2023/12/05